VBA – Dialog Box
Written by
Reviewed by
This article will demonstrate how to use Dialog Boxes in VBA.
Dialog Boxes are used to return information to the user. The most common dialog box that is used is the Message Box, but we can also use the Input Box.
Message Box
Syntax
The syntax of the Message Box is as follows:
Argument |
Explanation |
Prompt |
Message returned to the user – required |
Buttons |
Visual Basic buttons – defaults to OK if omitted |
Title |
Title of Message box – optional |
HelpFile |
Helpfile – optional |
Context |
Context – optional |
Simple Message Box
A simple message box just requires the prompt – the message you are returning to your user. The OK button will automatically be displayed.
Sub SimpleBox ()
MsgBox "Hello everybody!"
End Sub
YesNo Message Box
Adding in buttons can make you message box more flexible.
We can select the type of box from a drop down list of VB constants.
Sub MessageBox ()
Dim Result As Integer
Result = MsgBox("Are you sure you want to do that?", vbYesNo)
If Result = vbYes Then
MsgBox ("You do want to do that")
Else
MsgBox ("You do not want to do that")
End If
End Sub
As well as the Yes/No button, we can icons to display in our message box to indicate to the user what type of message is being displayed.
Sub MessageBox ()
Dim Result As Integer
Result = MsgBox("Are you sure you want to do that?", vbYesNo + vbQuestion)
If Result = vbYes Then
MsgBox "You do want to do that", vbInformation
Else
MsgBox "You do not want to do that", vbCritical
End If
End Sub
NOTE: if you do not select a button constant, but do select an information constant, you need to omit the brackets from around the message box arguments.
Adding a Title
We can also fill in the title argument.
Sub MessageBox ()
Dim Result As Integer
Result = MsgBox("Are you sure you want to do that?", vbYesNo + vbQuestion, "LET'S DO SOMETHING!")
If Result = vbYes Then
MsgBox "You do want to do that", vbInformation, "YES PLEASE"
Else
MsgBox "You do not want to do that", vbCritical, "NO THANKS"
End If
End Sub
For a more detailed article about Message Boxes, click here.
Input Box
An input box is a Dialog Box that asks the user a question, and returns a result.
Sub TestInputBox ()
Dim Result As String
Result = InputBox("What do you want to do?", "LET'S DO SOMETHING", "Enter your input text HERE")
MsgBox Result
End Sub
For a more detailed article about Input Boxes, click here.
VBA Coding Made Easy
Stop searching for VBA code online. Learn more about AutoMacro - A VBA Code Builder that allows beginners to code procedures from scratch with minimal coding knowledge and with many time-saving features for all users!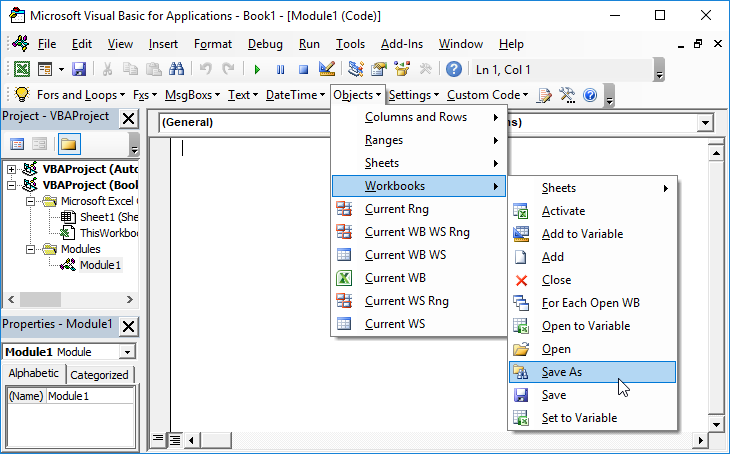
Learn More!